In software engineering, the decorator pattern is a structural design pattern that allows behavior to be added to an individual object, dynamically, without affecting the behavior of other objects from the same class.
The decorator pattern is often used to extend the functionality of a class without modifying the class itself. This can be useful when you want to add new functionality to a class without breaking existing code.
The decorator pattern can be implemented in Spring MVC by using the @Decorator annotation. The @Decorator annotation can be applied to a class to indicate that it is a decorator for another class.
## Spring MVC Decorator PatternThe decorator pattern is a structural design pattern that allows behavior to be added to an individual object, dynamically, without affecting the behavior of other objects from the same class.
Here are 3 important points about the spring MVC decorator pattern:
- It is implemented using the `@Decorator` annotation.
- It can be used to extend the functionality of a class without modifying the class itself.
- It is a powerful tool for creating flexible and extensible applications.
The decorator pattern is a valuable tool for Java developers. It can be used to create flexible and extensible applications that are easy to maintain.
### It is implemented using the `@Decorator` annotation. The `@Decorator` annotation is used to indicate that a class is a decorator for another class. The `@Decorator` annotation can be applied to any class that implements the `org.springframework.web.servlet.mvc.WebContentInterceptor` interface. When a class is annotated with the `@Decorator` annotation, Spring MVC will automatically wrap instances of that class around instances of the decorated class. This allows the decorator class to intercept and modify requests and responses that are processed by the decorated class. Decorators can be used to add a variety of functionality to a class, such as: * Logging * Security * Caching * Validation Decorators are a powerful tool for extending the functionality of Spring MVC applications. They can be used to add new features to applications without modifying the underlying code. Here is an example of a simple decorator class: ```java @Decorator public class LoggingDecorator implements WebContentInterceptor { @Override public void preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("Request: " + request.getRequestURI()); } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out.println("Response: " + response.getStatus()); } } ``` This decorator class can be used to log all requests and responses that are processed by the decorated class. To use the decorator class, simply add it to the Spring MVC configuration: ```java @Configuration public class WebMvcConfig { @Bean public LoggingDecorator loggingDecorator() { return new LoggingDecorator(); } } ``` Once the decorator class is added to the configuration, Spring MVC will automatically wrap instances of the decorated class around instances of the decorator class. ## Paragraph after details Decorators are a powerful tool for extending the functionality of Spring MVC applications. They can be used to add new features to applications without modifying the underlying code.### It can be used to extend the functionality of a class without modifying the class itself. One of the key benefits of the decorator pattern is that it allows you to extend the functionality of a class without modifying the class itself. This is important because it allows you to add new functionality to a class without breaking existing code. For example, let's say you have a class called `Customer`. The `Customer` class has a method called `getName()` that returns the name of the customer. Now, let's say you want to add a new method to the `Customer` class that returns the customer's full name. You could modify the `Customer` class to add the new method, but this would break any existing code that relies on the `Customer` class. Instead, you can use a decorator class to add the new method to the `Customer` class. The decorator class would wrap around the `Customer` class and provide the new method. Here is an example of a decorator class that adds a new method to the `Customer` class: ```java public class CustomerDecorator implements Customer { private Customer customer; public CustomerDecorator(Customer customer) { this.customer = customer; } @Override public String getName() { return customer.getName(); } public String getFullName() { return customer.getName() + " " + customer.getLastName(); } } ``` This decorator class can be used to add the `getFullName()` method to the `Customer` class without modifying the `Customer` class itself. To use the decorator class, simply wrap an instance of the `Customer` class with an instance of the decorator class: ```java Customer customer = new Customer(); CustomerDecorator decorator = new CustomerDecorator(customer); String fullName = decorator.getFullName(); ``` The decorator pattern is a powerful tool for extending the functionality of classes without modifying the classes themselves. This makes it a valuable tool for creating flexible and extensible applications. ## Paragraph after details Decorators can be used to add a variety of functionality to a class, such as: * Logging * Security * Caching * Validation Decorators are a powerful tool for extending the functionality of Spring MVC applications. They can be used to add new features to applications without modifying the underlying code.### It is a powerful tool for creating flexible and extensible applications. The decorator pattern is a powerful tool for creating flexible and extensible applications. Here are a few reasons why:Decorators can be used to add new functionality to a class without modifying the class itself. This makes it easy to add new features to applications without breaking existing code.
- Decorators can be easily added and removed.
Decorators are not hard-coded into a class. This means that they can be easily added and removed as needed. This makes it easy to experiment with different combinations of decorators to find the best solution for a particular application.
- Decorators can be used to create hierarchical relationships between classes.
Decorators can be used to create a hierarchy of classes, where each class adds new functionality to the class below it. This can make it easier to organize and maintain complex applications.
- Decorators can be used to create more flexible and reusable code.
Decorators can be used to create more flexible and reusable code. For example, a decorator can be used to add logging to a class. This decorator can then be reused in other classes that need logging.
- Decorators can be used to create more extensible applications.
Decorators can be used to create more extensible applications. For example, a decorator can be used to add a new feature to a class. This decorator can then be added to any class that needs the new feature.
The decorator pattern is a valuable tool for Java developers. It can be used to create flexible, extensible, and reusable applications.
### FAQHere are some frequently asked questions about the Spring MVC decorator pattern:
Question 1: What is the decorator pattern?
Answer: The decorator pattern is a structural design pattern that allows behavior to be added to an individual object, dynamically, without affecting the behavior of other objects from the same class.
Question 2: How is the decorator pattern implemented in Spring MVC?
Answer: The decorator pattern is implemented in Spring MVC using the `@Decorator` annotation. The `@Decorator` annotation can be applied to a class to indicate that it is a decorator for another class.
Question 3: What are some of the benefits of using the decorator pattern?
Answer: The decorator pattern offers several benefits, including: - It can be used to extend the functionality of a class without modifying the class itself. - It can be used to create flexible and extensible applications. - It can be used to create more reusable code.
Question 4: What are some examples of how the decorator pattern can be used in Spring MVC?
Answer: The decorator pattern can be used to add a variety of functionality to Spring MVC applications, such as: - Logging - Security - Caching - Validation
Question 5: How do I use the decorator pattern in my Spring MVC application?
Answer: To use the decorator pattern in your Spring MVC application, simply add the `@Decorator` annotation to the decorator class. Then, add the decorator class to the Spring MVC configuration.
Question 6: Where can I learn more about the decorator pattern?
Answer: There are a number of resources available online that can help you learn more about the decorator pattern. Some good starting points include the Spring MVC documentation and the JavaDoc for the `@Decorator` annotation.
In the next section, we will discuss some tips for using the decorator pattern in Spring MVC applications.
### TipsHere are a few tips for using the decorator pattern in Spring MVC applications:
Tip 1: Use the decorator pattern to add functionality to existing classes.
The decorator pattern can be used to add functionality to existing classes without modifying the classes themselves. This can be useful for adding features such as logging, security, and caching to existing classes.
Tip 2: Use the decorator pattern to create flexible and extensible applications.
The decorator pattern can be used to create flexible and extensible applications. By using decorators, you can easily add new functionality to applications without modifying the underlying code. This makes it easy to experiment with different combinations of decorators to find the best solution for a particular application.
Tip 3: Use the decorator pattern to create more reusable code.
The decorator pattern can be used to create more reusable code. For example, a decorator can be used to add logging to a class. This decorator can then be reused in other classes that need logging.
Tip 4: Use the decorator pattern to create more extensible applications.
The decorator pattern can be used to create more extensible applications. For example, a decorator can be used to add a new feature to a class. This decorator can then be added to any class that needs the new feature.
In the next section, we will discuss some of the benefits of using the decorator pattern in Spring MVC applications.
### Conclusion The decorator pattern is a powerful tool that can be used to create flexible, extensible, and reusable Spring MVC applications. By using decorators, you can easily add new functionality to applications without modifying the underlying code. This makes it easy to experiment with different combinations of decorators to find the best solution for a particular application. The decorator pattern is a valuable tool for Java developers. It can be used to create a wide variety of applications, from simple web applications to complex enterprise applications. If you are not familiar with the decorator pattern, I encourage you to learn more about it. ### Closing Message I hope this article has been helpful in providing you with a better understanding of the decorator pattern and how it can be used in Spring MVC applications. If you have any questions, please feel free to leave a comment below.
Diy spring room decor bethany mota
Easy diy spring room decor
Spring decorations factory

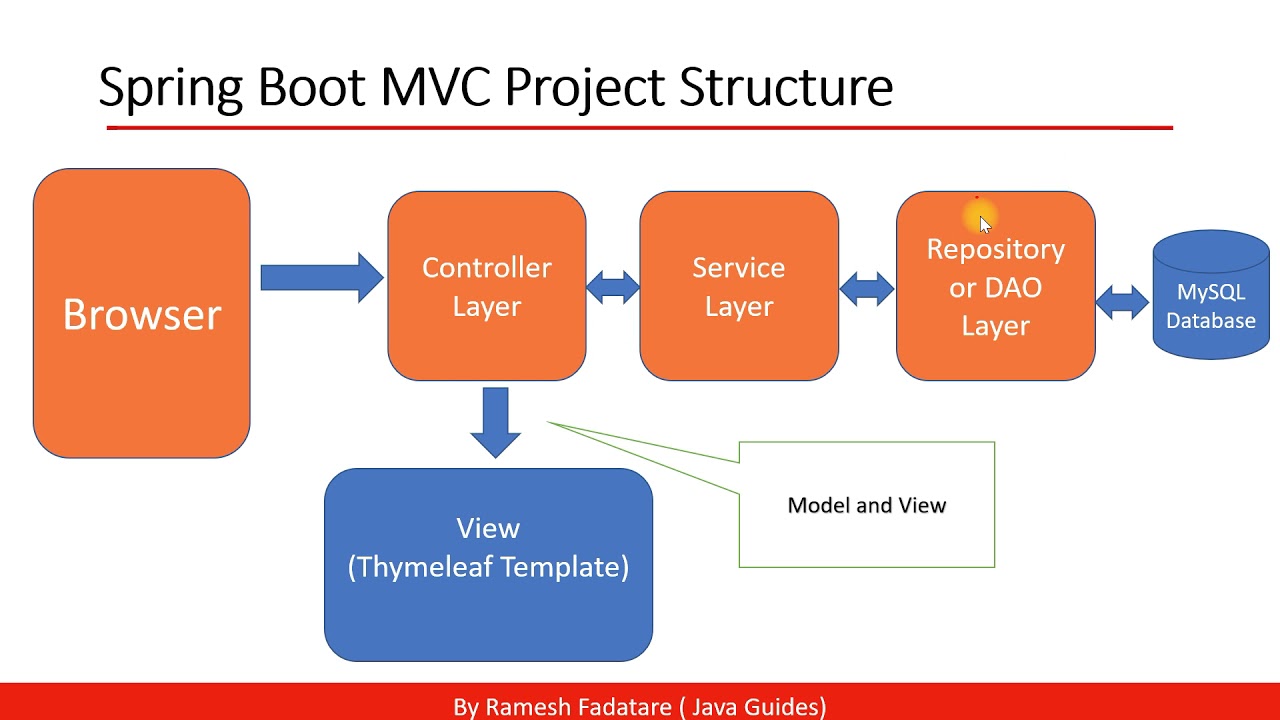
